Endpoints
The Proxy accepts HTTP requests on the following endpoints.
CDN Proxy
The CDN proxy endpoint's purpose is to forward the underlying config JSON to other ConfigCat SDKs used by your application.
Regarding the config JSON's schema changes introduced recently (v6
), the v0.3.X
and newer Proxy versions are providing the new schema on the CDN proxy endpoint.
This new config JSON schema is only supported from certain SDK versions listed in the support table below.
Those SDKs that are older than the listed versions are incompatible with v0.3.X
, and can be used only with v0.2.X
or older Proxy versions.
- Proxy version 0.3.X or newer
- Proxy version 0.2.X or older
GETOPTIONS/configuration-files/configcat-proxy/{sdkId}/config_v6.json
This endpoint is mainly used by ConfigCat SDKs to retrieve all required data for feature flag evaluation.
Route parameters:
sdkId
: The SDK identifier that uniquely identifies an SDK within the Proxy.
Responses:
- 200: The
config.json
file is downloaded successfully. - 204: In response to an
OPTIONS
request. - 304: The
config.json
file isn't modified based on theEtag
sent in theIf-None-Match
header. - 400: The
sdkId
is missing. - 404: The
sdkId
is pointing to a non-existent SDK.
SDK Usage
In order to let a ConfigCat SDK use the Proxy, you have to set the SDK's baseUrl
parameter to point to the Proxy's host.
Also, you have to pass the SDK identifier prefixed with configcat-proxy/
as the SDK key.
So, let's assume you set up the Proxy with the following SDK option:
- YAML
- Environment variables
sdks:
my_sdk:
key: "<your-sdk-key>"
CONFIGCAT_SDKS={"my_sdk":"<your-sdk-key>"}
The SDK's initialization that works with the Proxy will look like this:
import * as configcat from "configcat-js";
var configCatClient = configcat.getClient(
"configcat-proxy/my_sdk", // SDK identifier as SDK key
configcat.PollingMode.AutoPoll,
{ baseUrl: "http(s)://localhost:8050" } // Proxy URL
);
Supported SDK Versions
The following SDK versions are supported by the >=v0.3.X
Proxy's CDN endpoint:
SDK | Version |
---|---|
.NET | >=v9.0.0 |
JS | >=v9.0.0 |
JS SSR | >=v8.0.0 |
React | >=v4.0.0 |
Node | >=v11.0.0 |
Python | >=v9.0.3 |
Go | >=v9.0.0 |
C++ | >=v4.0.0 |
Dart | >=v4.0.0 |
Elixir | >=v4.0.0 |
Java | >=v9.0.0 |
Android | >=v10.0.0 |
Kotlin | >=v3.0.0 |
PHP 8.1+ | >=v9.0.0 |
PHP 7.1+ | >=v3.0.0 |
Ruby | >=v8.0.0 |
Swift | >=v11.0.0 |
GETOPTIONS/configuration-files/{sdkId}/config_v5.json
This endpoint is mainly used by ConfigCat SDKs to retrieve all required data for feature flag evaluation.
Route parameters:
sdkId
: The SDK identifier that uniquely identifies an SDK within the Proxy.
Responses:
- 200: The
config.json
file is downloaded successfully. - 204: In response to an
OPTIONS
request. - 304: The
config.json
file isn't modified based on theEtag
sent in theIf-None-Match
header. - 400: The
sdkId
is missing. - 404: The
sdkId
is pointing to a non-existent SDK.
SDK Usage
In order to let a ConfigCat SDK use the Proxy, you have to set the SDK's baseUrl
parameter to point to the Proxy's host.
Also, you have to pass the SDK identifier as the SDK key.
So, let's assume you set up the Proxy with the following SDK option:
- YAML
- Environment variables
sdks:
my_sdk:
key: "<your-sdk-key>"
CONFIGCAT_SDKS={"my_sdk":"<your-sdk-key>"}
The SDK's initialization that works with the Proxy will look like this:
import * as configcat from "configcat-js";
var configCatClient = configcat.getClient(
"my_sdk", // SDK identifier as SDK key
configcat.PollingMode.AutoPoll,
{ baseUrl: "http(s)://localhost:8050" } // Proxy URL
);
Supported SDK Versions
The following SDK versions are supported by the <=v0.2.X
Proxy's CDN endpoint:
SDK | Version |
---|---|
.NET | <=v8.2.0 |
JS | <=v8.1.1 |
JS SSR | <=v7.1.1 |
React | <=v3.1.1 |
Node | <=v10.1.1 |
Python | <=v8.0.1 |
C++ | <=v3.1.1 |
Dart | <=v3.0.0 |
Elixir | <=v3.0.0 |
Go | <=v8.0.1 |
Java | <=v8.4.0 |
Android | <=v9.1.1 |
Kotlin | <=2.0.0 |
PHP | <=v8.1.0 |
Ruby | <=v7.0.0 |
Swift | <=v10.0.0 |
Available Options
The following CDN Proxy related options are available:
Option | Default | Description |
---|---|---|
| true | Turns the hosting of the CDN proxy endpoint on/off. This endpoint can be used by other ConfigCat SDKs in your applications. |
| true | Turns the sending of CORS headers on/off. It can be used to restrict access to specific domains. By default, the Proxy allows each origin by setting the Access-Control-Allow-Origin response header to the request's origin. You can override this functionality by restricting the allowed origins with the allowed_origins or allowed_origins_regex options. |
| - | List of allowed CORS origins. When it's set, the Proxy will include only that origin in the Access-Control-Allow-Origin response header which matches the request's Origin .When there's no matching request origin and the allowed_origins_regex option is not set, the Proxy will set the Access-Control-Allow-Origin response header to the first item in the allowed origins list. |
| - | List of regex patterns used to match allowed CORS origins. When it's set, the Proxy will match the request's |
| - | Required when the previous patterns option is set. It's value is used in the Access-Control-Allow-Origin header when an incoming request's Origin doesn't match with any previously configured regex patterns. |
| - | Additional headers that must be sent back on each CDN proxy endpoint response. |
API
The API endpoints are for server side feature flag evaluation.
POSTOPTIONS/api/{sdkId}/eval
This endpoint evaluates a single feature flag identified by a key
with the given User Object.
Route parameters:
sdkId
: The SDK identifier that uniquely identifies an SDK within the Proxy.
Request body:
{
"key": "<feature-flag-key>",
"user": {
"Identifier": "<user-id>",
"Rating": 4.5,
"Roles": ["Role1","Role2"],
// any other attribute
}
}
The type of the user
object's fields can only be string
, number
, or string[]
.
Responses:
- 200: The feature flag evaluation finished successfully.
- 204: In response to an
OPTIONS
request. - 400: The
sdkId
or thekey
from the request body is missing. - 404: The
sdkId
is pointing to a non-existent SDK.
{
"value": <evaluated-value>,
"variationId": "<variation-id>"
}
POSTOPTIONS/api/{sdkId}/eval-all
This endpoint evaluates all feature flags with the given User Object.
Route parameters:
sdkId
: The SDK identifier that uniquely identifies an SDK within the Proxy.
Request body:
{
"key": "<feature-flag-key>",
"user": {
"Identifier": "<user-id>",
"Rating": 4.5,
"Roles": ["Role1","Role2"],
// any other attribute
}
}
The type of the user
object's fields can only be string
, number
, or string[]
.
Responses:
- 200: The evaluation of all feature flags finished successfully.
- 204: In response to an
OPTIONS
request. - 400: The
sdkId
is missing. - 404: The
sdkId
is pointing to a non-existent SDK.
{
"feature-flag-key-1": {
"value": <evaluated-value>,
"variationId": "<variation-id>"
},
"feature-flag-key-2": {
"value": <evaluated-value>,
"variationId": "<variation-id>"
}
}
POSTOPTIONS/api/{sdkId}/refresh
This endpoint commands the underlying SDK to download the latest available config JSON.
Route parameters:
sdkId
: The SDK identifier that uniquely identifies an SDK within the Proxy.
Responses:
- 200: The refresh was successful.
- 204: In response to an
OPTIONS
request. - 400: The
sdkId
is missing. - 404: The
sdkId
is pointing to a non-existent SDK.
GETOPTIONS/api/{sdkId}/keys
This endpoint returns all feature flag keys belonging to the given SDK identifier.
Route parameters:
sdkId
: The SDK identifier that uniquely identifies an SDK within the Proxy.
Responses:
- 200: The keys are returned successfully.
- 204: In response to an
OPTIONS
request. - 400: The
sdkId
is missing. - 404: The
sdkId
is pointing to a non-existent SDK.
{
"keys": [
"feature-flag-key-1",
"feature-flag-key-1"
]
}
Available Options
The following API related options are available:
Option | Default | Description |
---|---|---|
| true | Turns the hosting of the API endpoints on/off. These endpoints can be used for server side feature flag evaluation. |
| true | Turns the sending of CORS headers on/off. It can be used to restrict access to specific domains. By default, the Proxy allows each origin by setting the Access-Control-Allow-Origin response header to the request's origin. You can override this functionality by restricting the allowed origins with the allowed_origins or allowed_origins_regex options. |
| - | List of allowed CORS origins. When it's set, the Proxy will include only that origin in the Access-Control-Allow-Origin response header which matches the request's Origin .When there's no matching request origin and the allowed_origins_regex option is not set, the Proxy will set the Access-Control-Allow-Origin response header to the first item in the allowed origins list. |
| - | List of regex patterns used to match allowed CORS origins. When it's set, the Proxy will match the request's |
| - | Required when the previous patterns option is set. It's value is used in the Access-Control-Allow-Origin header when an incoming request's Origin doesn't match with any previously configured regex patterns. |
| - | Additional headers that must be sent back on each API endpoint response. |
| - | Additional headers that must be on each request sent to the API endpoints. If the request doesn't include the specified header, or the values are not matching, the Proxy will respond with a 401 HTTP status code. |
SSE
The SSE endpoint allows you to subscribe for feature flag value changes through Server-Sent Events connections.
GETOPTIONS/sse/{sdkId}/eval/{data}
This endpoint subscribes to a single flag's changes. Whenever the watched flag's value changes, the Proxy sends the new value to each connected client.
Route parameters:
sdkId
: The SDK identifier that uniquely identifies an SDK within the Proxy.data
: Thebase64
encoded input data for feature flag evaluation that must contain the feature flag's key and a User Object.
Responses:
- 200: The SSE connection established successfully.
- 204: In response to an
OPTIONS
request. - 400: The
sdkId
,data
, or thekey
attribute ofdata
is missing. - 404: The
sdkId
is pointing to a non-existent SDK.
{
"value": <evaluated-value>,
"variationId": "<variation-id>"
}
Example:
const rawData = {
key: "<feature-flag-key>",
user: { // field types can only be `string`, `number`, or `string[]`.
Identifier: "<user-id>",
Rating: 4.5,
Roles: ["Role1","Role2"],
// any other attribute
}
}
const data = btoa(JSON.stringify(rawData))
const evtSource = new EventSource("http(s)://localhost:8050/sse/my_sdk/eval/" + data);
evtSource.onmessage = (event) => {
console.log(event.data); // {"value":<evaluated-value>,"variationId":"<variation-id>"}
};
GETOPTIONS/sse/{sdkId}/eval-all/{data}
This endpoint subscribes to all feature flags' changes behind the given SDK identifier. When any of the watched flags' value change, the Proxy sends its new value to each connected client.
Route parameters:
sdkId
: The SDK identifier that uniquely identifies an SDK within the Proxy.data
: Optional. Thebase64
encoded input data for feature flag evaluation that contains a User Object.
Responses:
- 200: The SSE connection established successfully.
- 204: In response to an
OPTIONS
request. - 400: The
sdkId
is missing. - 404: The
sdkId
is pointing to a non-existent SDK.
{
"feature-flag-key-1": {
"value": <evaluated-value>,
"variationId": "<variation-id>"
},
"feature-flag-key-2": {
"value": <evaluated-value>,
"variationId": "<variation-id>"
}
}
Example:
const rawData = {
user: { // field types can only be `string`, `number`, or `string[]`.
Identifier: "<user-id>",
Rating: 4.5,
Roles: ["Role1","Role2"],
// any other attribute
}
}
const data = btoa(JSON.stringify(rawData))
const evtSource = new EventSource("http(s)://localhost:8050/sse/my_sdk/eval-all/" + data);
evtSource.onmessage = (event) => {
console.log(event.data); // {"feature-flag-key":{"value":<evaluated-value>,"variationId":"<variation-id>"}}
};
Available Options
The following SSE related options are available:
Option | Default | Description |
---|---|---|
| true | Turns the hosting of the SSE endpoint on/off, This endpoint can be used to stream feature flag value changes. |
| true | Turns the sending of CORS headers on/off. It can be used to restrict access to specific domains. By default, the Proxy allows each origin by setting the Access-Control-Allow-Origin response header to the request's origin. You can override this functionality by restricting the allowed origins with the allowed_origins or allowed_origins_regex options. |
| - | List of allowed CORS origins. When it's set, the Proxy will include only that origin in the Access-Control-Allow-Origin response header which matches the request's Origin .When there's no matching request origin and the allowed_origins_regex option is not set, the Proxy will set the Access-Control-Allow-Origin response header to the first item in the allowed origins list. |
| - | List of regex patterns used to match allowed CORS origins. When it's set, the Proxy will match the request's |
| - | Required when the previous patterns option is set. It's value is used in the Access-Control-Allow-Origin header when an incoming request's Origin doesn't match with any previously configured regex patterns. |
| - | Additional headers that must be sent back on each SSE endpoint response. |
| warn | The verbosity of the SSE related logs. Possible values: error , warn , info or debug . |
Webhook
Through the webhook endpoint, you can notify the Proxy about the availability of new feature flag evaluation data. Also, with the appropriate SDK options, the Proxy can validate the signature of each incoming webhook request.
GETPOST/hook/{sdkId}
Notifies the Proxy that the SDK with the given SDK identifier must refresh its config JSON to the latest version.
Route parameters:
sdkId
: The SDK identifier that uniquely identifies an SDK within the Proxy.
Responses:
- 200: The Proxy accepted the notification.
- 400: The
sdkId
is missing or the webhook signature validation failed. - 404: The
sdkId
is pointing to a non-existent SDK.
ConfigCat Dashboard
You can set up webhooks to invoke the Proxy on the Webhooks page of the ConfigCat Dashboard.
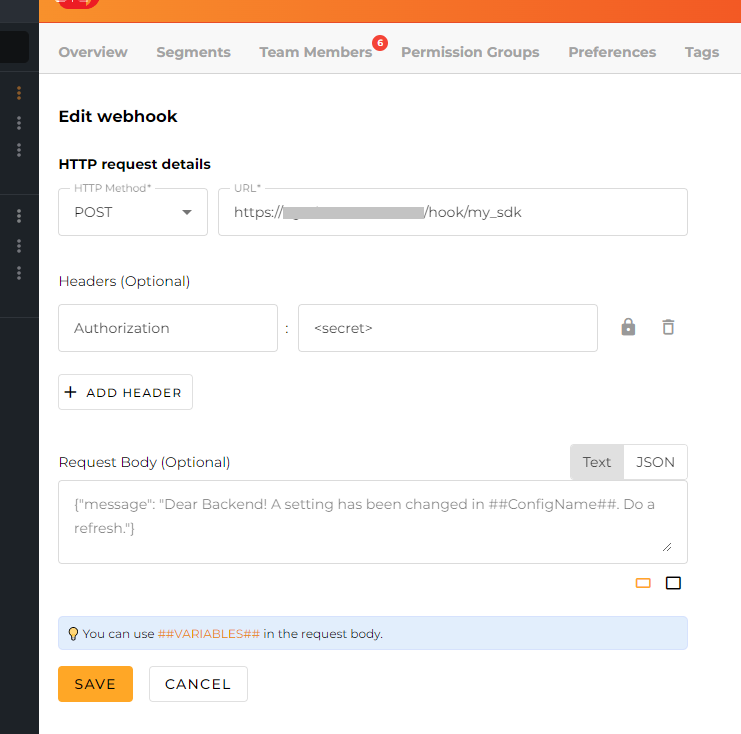
Available Options
The following webhook related options are available:
Option | Default | Description |
---|---|---|
| true | Turns the hosting of the Webhook endpoint on/off. This endpoint can be used to notify the Proxy about the availability of new feature flag evaluation data. |
| - | Basic authentication user. The basic authentication webhook header can be set on the Webhooks page of the ConfigCat Dashboard. |
| - | Basic authentication password. The basic authentication webhook header can be set on the Webhooks page of the ConfigCat Dashboard. |
| - | Additional headers that ConfigCat must send with each request to the Webhook endpoint. Webhook headers can be set on the Webhooks page of the ConfigCat Dashboard. |